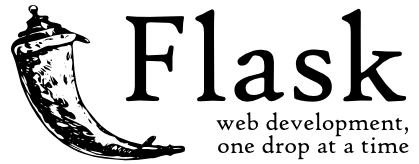
The “micro” in microframework means Flask aims to keep the core simple but extensible. Flask won’t make many decisions for you, such as what database to use. Everything else is up to you, so that Flask can be everything you need and nothing you don’t.
By default, Flask does not include a database abstraction layer, form validation or anything else where different libraries already exist that can handle that. Instead, Flask supports extensions to add such functionality to your application as if it was implemented in Flask itself. Numerous extensions provide database integration, form validation, upload handling, various open authentication technologies, and more. Flask may be “micro”, but it’s ready for production use on a variety of needs.
Do you have the latest Python?
Make sure to have or install the latest version of Python; Flask supports Python 3.6 and newer. async support in Flask requires Python 3.7+ for contextvars.ContextVar.
Dependencies
The following will be automatically installed when you install Flask.
- Werkzeug
- Jinja
- MarkupSafe
- ItsDangerous
- Click
The following will not be automatically installed because they are optional dependencies. Flask will detect and use them if you install them.
- Blinker
- python-dotenv
- Watchdog
Virtual environments
It is highly recommended that you use a virtual environment to manage the dependencies for your project, both in development and in production. Virtual environments are independent groups of Python libraries, one for each project. Packages installed for one project will not affect other projects or the operating system’s packages.
How to create an environment?
Create a folder for your project then create a venv folder within.
- macOS/Linux
$ mkdir myproject $ cd myproject $ python3 -m venv venv
- Windows
> mkdir myproject > cd myproject > py -3 -m venv venv
How to activate an environment?
You need to activate the environment before you start working on your project. Activate the environment with this command:
- macOS/Linux
$ . venv/bin/activate
- Windows
> venv\Scripts\activate
Your shell prompt will change to show the name of the activated environment.
Finally, you can install Flask
Within the activated environment, use the following command to install Flask:
$ pip install Flask
Flask is now installed.
That eager to start? This section is a good introduction to Flask. Be sure to read the Installation before proceeding.
A Mini App
It as basic as this:
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello_world():
return "Hello, World!"
Code explanation
- First we imported the Flask class. An instance of this class will be our WSGI application.
- Next we create an instance of this class. The first argument is the name of the application’s module or package.
__name__
is a convenient shortcut for this that is appropriate for most cases. This is needed so that Flask knows where to look for resources such as templates and static files. - We then use the route() decorator to tell Flask what URL should trigger our function.
- The function returns the message we want to display in the user’s browser. The default content type is HTML, so HTML in the string will be rendered by the browser.
Save it as greeting.py or something similar. Make sure to not call your application flask.py because this would conflict with Flask itself.
To run the application, use the flask command or python -m flask. Before you can do that you need to tell your terminal the application to work with by exporting the FLASK_APP
environment variable:
- Bash
$ export FLASK_APP=hello $ flask run * Running on http://127.0.0.1:5000/
- CMD
> set FLASK_APP=hello > flask run * Running on http://127.0.0.1:5000/
- Powershell
> $env:FLASK_APP = "hello" > flask run * Running on http://127.0.0.1:5000/
Modern web applications use meaningful URLs to help users. Users are more likely to like a page and come back if the page uses a meaningful URL they can remember and use to directly visit a page.
Use the route()
decorator to bind a function to a URL.
@app.route('/')
def index():
return 'Index Page'
@app.route('/hello')
def hello():
return 'Hello, World'
You can do more! You can make parts of the URL dynamic and attach multiple rules to a function.
To redirect a user to another endpoint, use the redirect()
function; to abort a request early with an error code, use the abort()
function:
from flask import abort, redirect, url_for
@app.route('/')
def index():
return redirect(url_for('login'))
@app.route('/login')
def login():
abort(401)
this_is_never_executed()
This is a rather pointless example because a user will be redirected from the index to a page they cannot access (401 means access denied) but it shows how that works.
By default a black and white error page is shown for each error code. If you want to customize the error page, you can use the errorhandler()
decorator:
from flask import render_template
@app.errorhandler(404)
def page_not_found(error):
return render_template('page_not_found.html'), 404
Note the 404
after the render_template()
call. This tells Flask that the status code of that page should be 404 which means not found. By default 200 is assumed which translates to: all went well.